AnĂșncios
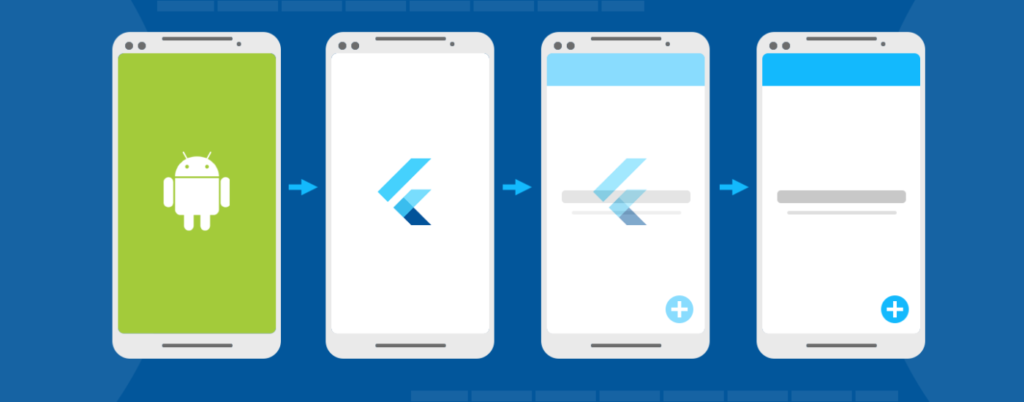
How to develop an app with the Flutter Framework: step-by-step
Developing a mobile app can be an exciting and rewarding experience. With the Flutter framework, you can create cross-platform apps that run seamlessly on iOS and Android devices.
Whether you’re a beginner or an experienced developer, this step-by-step guide will walk you through the process of developing an app using Flutter.
AnĂșncios
What is Flutter?
Flutter is an open-source UI software development kit (SDK) created by Google. It allows developers to build natively compiled applications for mobile, web, and desktop from a single codebase.
Flutter uses the Dart programming language and provides a rich set of customizable widgets, enabling developers to create beautiful and responsive user interfaces.
AnĂșncios
Setting up the development environment
Before getting started with Flutter app development, you need to set up your development environment. Here’s how:
Installing Flutter SDK
To install the Flutter SDK, follow these steps:
- Visit the Flutter website (https://flutter.dev/) and download the Flutter SDK for your operating system.
- Extract the downloaded file to a location on your computer.
- Add the Flutter SDK’s bin directory to your system’s PATH variable.
Installing an IDE (Integrated Development Environment)
You can choose any IDE for Flutter development, but two popular options are Android Studio and Visual Studio Code (VS Code). Here’s how to install them:
Android Studio:
- Download and install Android Studio from the official website (https://developer.android.com/studio).
- Open Android Studio and install the Flutter and Dart plugins.
- Configure the Flutter SDK path in Android Studio.
Visual Studio Code:
- Download and install Visual Studio Code from the official website (https://code.visualstudio.com/).
- Open Visual Studio Code and install the Flutter and Dart extensions.
- Configure the Flutter SDK path in Visual Studio Code.
Configuring the IDE for Flutter development
After installing the IDE, you need to configure it for Flutter development:
- Open the IDE and install any additional plugins or extensions recommended for Flutter development.
- Set up the Dart SDK path in the IDE’s preferences or settings.
- Install any required emulators or simulators for testing your app.
Now that your development environment is set up, let’s move on to creating a new Flutter project.
Creating a new Flutter project
To create a new Flutter project, follow these steps:
Using the Flutter command-line interface (CLI)
- Open a terminal or command prompt.
- Run the following command to create a new Flutter project:
- Change directory to the newly created project
Creating a basic Flutter app structure
A Flutter project has a predefined directory structure. Here are the key directories and files you’ll work with:
- lib: Contains the Dart code for your app.
- test: Contains the test files for your app.
- android: Contains the Android-specific configuration files.
- ios: Contains the iOS-specific configuration files.
Now that we have set up our project, let’s explore Flutter widgets in the next section.
Understanding Flutter widgets
Widgets are the building blocks of Flutter UI. They are UI elements, such as buttons, text fields, and containers, that are assembled together to create the user interface of your app. Flutter provides a wide range of pre-built widgets, and you can also create your own custom widgets.
Exploring the widget tree
In Flutter, widgets are organized in a hierarchical structure called the “widget tree.” The widget tree represents the visual hierarchy of your app’s UI. Each widget can have child widgets, and together they form a tree-like structure.
Stateless vs. stateful widgets
In Flutter, there are two main types of widgets: stateless widgets and stateful widgets.
A stateless widget is immutable and doesn’t maintain any internal state. It represents UI elements that don’t change over time, such as labels or icons.
A stateful widget, on the other hand, can hold internal state that can change over time. For example, a form that accepts user input would typically be implemented as a stateful widget.
Now that we have a basic understanding of Flutter widgets, let’s move on to building the user interface of our app.
Building the user interface
The user interface is a crucial aspect of any app. Flutter provides a rich set of pre-built widgets that you can use to create your app’s UI. Let’s explore how to build the user interface using Flutter widgets.
Using pre-built Flutter widgets
Flutter offers a wide range of pre-built widgets that you can use out of the box. Some commonly used widgets include:
- Container: A widget that provides a rectangular visual layout.
- Text: A widget for displaying text.
- Button: Widgets for creating buttons with different styles.
- Image: A widget for displaying images.
Customizing widgets
While pre-built widgets are useful, you often need to customize them to match your app’s specific requirements. Flutter allows you to customize widgets by modifying their properties and applying styles.
For example, you can change the text color and font size of a Text widget or add a background color to a Container widget. By combining and customizing widgets, you can create unique and visually appealing UIs.
Creating responsive layouts
In today’s mobile world, it’s essential to create responsive layouts that adapt to different screen sizes and orientations. Flutter provides various techniques for creating responsive layouts, such as using MediaQuery and LayoutBuilder widgets.
You can use MediaQuery to retrieve information about the app’s device and adjust the UI accordingly. LayoutBuilder, on the other hand, allows you to build layouts based on the parent widget’s constraints.
Now that we have built our app’s UI, let’s move on to handling user input and interactions.
Handling user input and interactions
User input and interactions play a vital role in app development. Flutter provides a set of widgets and techniques for handling user input, button presses, text input, gestures, and animations.
Adding buttons and handling button presses
Buttons are a common UI element in mobile apps. Flutter provides different types of buttons, such as RaisedButton, FlatButton, and IconButton. You can attach event handlers to buttons to perform actions when they are pressed.
For example, to navigate to a new screen when a button is pressed, you can use the Navigator widget to push a new route onto the navigation stack.
Text input and form validation
Text input is another crucial aspect of many apps. Flutter provides the TextField widget for capturing user input. You can validate user input using various validation techniques, such as regular expressions or custom validation functions.
Flutter also provides Form widgets that help in managing complex forms with multiple input fields. You can perform form validation and handle form submission using the Form widget.
Gestures and animations
Flutter has excellent support for gestures and animations, allowing you to create interactive and engaging user experiences. You can detect gestures like taps, swipes, and pinches using GestureDetector or specialized gesture recognizer widgets.
To create animations, you can use the Animation and AnimationController classes provided by Flutter. With these classes, you can define animations for various UI elements, such as fading in and out or sliding transitions.
Now that we know how to handle user input and interactions, let’s move on to managing app state.
Managing app state
App state management is a critical aspect of Flutter app development. As your app grows in complexity, you need to manage the state of various UI elements and data.
Introduction to app state management
App state refers to the data that is shared and accessed by different parts of your app. Managing app state involves storing, updating, and synchronizing data across different widgets and screens.
Flutter offers various state management solutions, ranging from built-in solutions to third-party packages. The choice of state management solution depends on the complexity of your app and your preferences.
Using built-in state management solutions
Flutter provides built-in state management solutions, such as setState and InheritedWidget. These solutions work well for small to medium-sized apps with relatively simple state management requirements.
setState is a basic mechanism that allows you to update the state of a widget and trigger a rebuild of the UI. InheritedWidget is a widget that allows you to share state across the widget tree without passing it explicitly to each widget.
Implementing a third-party state management solution
For larger apps with more complex state management needs, you can consider using third-party state management packages like Provider, Riverpod, or MobX. These packages provide more advanced features, such as dependency injection, reactive programming, and scoped models.
The choice of a state management solution depends on your app’s requirements and your familiarity with the package.
Now that we understand app state management, let’s explore testing and debugging Flutter apps.
Testing and debugging
Testing and debugging are essential steps in the app development process. Flutter provides tools and techniques for testing and debugging your app to ensure it functions as expected.
Running tests in Flutter
Flutter has built-in support for unit testing, widget testing, and integration testing. You can write tests using the Flutter testing framework and run them using the Flutter test command.
Unit tests help verify the correctness of individual functions or classes, while widget tests allow you to test UI components and their interactions. Integration tests are used to test the behavior of multiple components working together.
Debugging techniques and tools
When developing apps, it’s common to encounter bugs and issues. Flutter provides several debugging techniques and tools to help you identify and fix problems.
You can use Flutter’s debugging tools, such as the Dart Observatory and the Flutter DevTools, to inspect the app’s runtime behavior, view logs, and analyze performance. Flutter also integrates with popular IDEs like Android Studio and Visual Studio Code, providing a seamless debugging experience.
Now that we know how to test and debug Flutter apps, let’s move on to publishing the app.
Conclusion
Developing an app with the Flutter framework provides a powerful and efficient way to create cross-platform applications. In this article, we covered the step-by-step process of developing an app using Flutter, from setting up the development environment to publishing the app.
We explored important concepts like Flutter widgets, handling user input and interactions, managing app state, testing and debugging, optimizing app performance, and following best practices.
By following this guide and harnessing the capabilities of the Flutter framework, you can create high-quality, feature-rich apps that delight users on both iOS and Android platforms.